Hello guys, its every developer’s dream to get a SDE job on FAANG company. I dreamed it too and having gone through interview on Google, Microsoft and Amazon, I know a thing or two about these interviews. Many developer fail these interview either because of data structures and algorithms or because of System Design. Even for experienced developer cracking a system design interview is not easy. It requires patience, perseverance and dedication to learn the intricacies of system design and acquire knowledge to crack interview.
Java67
Learn Java and Programming through articles, code examples, and tutorials for developers of all levels.
Top 8 Online Courses to Learn System Design and Software Architecture on Udemy (2025)
Hello friends, If you're aiming to master Software Architecture and System Design in 2025, you're in the right place. Whether you're preparing for tough technical interviews or simply want to become a better software engineer, understanding system design is a must. But let’s be honest—it’s also one of the most challenging topics to master. Many developers, including experienced ones, struggle with system design interviews—especially when applying to top tech companies like Google, Meta, Amazon, Apple, Microsoft, and Netflix (formerly known as FAANG, now MAANG).
10 Essential Object Oriented Concepts for Java Developers
Top 5 Java Performance Courses for Experienced Developers in 2025 - Best of Lot
Top 5 Free Udemy Courses to Learn Google Cloud Platform for Beginners in 2025 - Best of Lot
Tech Certification Practice Tests - Java +Spring + Data + AI + Cloud - All $9.99 (Limited Time)
Dear Students,
I hope this email finds you well and making progress on your tech certification journey in 2025!
As many of you have reached out asking for additional practice resources, I wanted to share some exciting news.
For a limited time only (until March 28th), all of my certification practice test courses on Udemy are available for just $9.99 each with the coupon code MARCH2024.
How to Fix Unsupported major.minor version 60.0, 59.0, 58.0, 57.0, 56.0, 55.0, 54, 0, 53.0, 52.00, 51.0 Error in Eclipse & Java
How to fix java.lang.OutOfMemoryError: unable to create new native thread [Solution]
10 Essential Spring MVC and REST Annotations with Examples for Java Programmers
How to solve java.lang.NoClassDefFoundError: org/springframework/beans/factory/SmartInitializingSingleton in Spring Boot [Solved]
I was trying to run a HelloWorld program using Spring Boot when I got this error:
Exception in thread "main" java.lang.IllegalStateException: Could not evaluate condition on org.springframework.boot.autoconfigure.PropertyPlaceholderAutoConfiguration#propertySourcesPlaceholderConfigurer due to internal class not found. This can happen if you are @ComponentScanning a springframework package (e.g. if you put a @ComponentScan in the default package by mistake)
at org.springframework.boot.autoconfigure.condition.SpringBootCondition.matches(SpringBootCondition.java:52)
at org.springframework.context.annotation.ConditionEvaluator.shouldSkip(ConditionEvaluator.java:92)
at org.springframework.context.annotation.ConfigurationClassBeanDefinitionReader.loadBeanDefinitionsForBeanMethod(ConfigurationClassBeanDefinitionReader.java:174)
at org.springframework.context.annotation.ConfigurationClassBeanDefinitionReader.loadBeanDefinitionsForConfigurationClass(ConfigurationClassBeanDefinitionReader.java:136)
Difference between @Component, @Controller, @Service, and @Repository in Spring
29 Spring Framework Interview Questions Answers for 5 years Experienced
Top 30 OOP (Object Oriented Programming) Interview Questions Answers in Java
12 Best Udemy and Educative React.js Courses for Beginners and Experienced in 2025
If you are a Web developer or someone passionate about web development and looking for some awesome courses to learn React, React.js, or React JS, a popular JavaScript framework to develop a component-based user interface then you have come to the right place.
Facebook’s React library has taken the front-end development world by storm. More and more people have started using React.js even in favor of Google’s Angular, another popular front-end development framework.
Well, I am not going into the classic debate of Angular vs React
as both frameworks have their advantage and disadvantages but if you
have chosen to learn React, you have made the right decision.
For those who don’t know what is React and Redux, let me tell you that React is a JavaScript library
that allows you to build complex GUI for single-page web applications
and mobile applications by re-using several small components.
For example, you build a component to display the header and image and reuse that to create a board of tasks.
Similarly, Redux
is another JavaScript library for managing application state. It is
most commonly used along with other JavaScript libraries like Angular
and React for developing modern-day front-end applications.
In the past, I have shared the 2025 React Developer RoadMap and some free courses to learn React, which helped a lot of my friends and readers and they asked me to also make a list of the best online React courses.
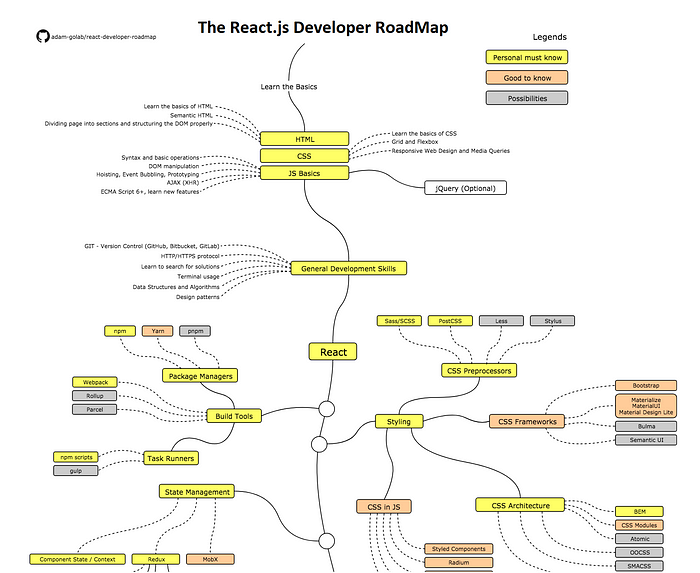
So, I will share some of the most popular courses to learn React and Redux frameworks to make user interface development easier.
If you are a front-end developer
you can use these courses to further enhance your skill and if you are a
back-end developer then also you can join this course to learn React
JS.
If you are thinking about why one should learn the React
library, I think knowledge of popular front-end libraries like Angular
and React is important for programmers who want to become full-stack
developers, and nowadays full-stack developers are in good demand.
So, learning React will also improve your chances of getting a job as a
web developer. Btw, if you are in a rush and don’t want to go through
the full list, you can always start with the React 16 — The Complete Guide by Max on Udemy. This is one of the best courses to learn React and I am also learning from the same course.
12 Best Udemy + Educative React JS Online Courses for Web Developers in 2025
If
you are wondering should learn to React now or later then let me tell
you that there is no better time to learn React than now as companies
like Facebook, Walmart, Uber, Airbnb, and Netflix are all using React in
production.
They’ve also made heavy investments in the React ecosystem, creating new tools and libraries to speed up and simplify React development
Since
everyone’s using React right now, from big to small companies, the
demand for React JS developers is very high, and more and more React
jobs are popping up every day.
Anyway, Without any further ado, here is my list of some of the best courses to learn React.js in 2025
1. Modern React with Redux (2025 Update)
This
is one of the best courses to learn React JS and Redux on Udemy. The
instructor Stephen Grider is one of the best instructors in the
JavaScript front-end development space with more than 230K students
enrolled in his courses like this one.
He is an expert in building complex JavaScript front ends for top corporations in the San Francisco Bay Area and that shows in this course.
The
course covers most of the React and Redux fundamental concepts like
fundamentals of React, JSX (React’s custom markup language), “props”,
“state”, eventing, etc, and also touches new topics like ES 6 and
advanced concepts like Babel and WebPack, which are important for full-stack web developers.
Here is the link to join this course — Modern React with Redux (2025 Update)

You will also learn about writing clean code using JSX and testing your React application, which is very important for a professional web developer.
As far as the course quality is concerned. Stephen Grider is a Great mentor. He is experienced and has a deep understanding of subjects like React and building complex front-ends using JavaScript, and most importantly, he explains each lecture in-depth.
He also keeps his course up-to-date. In short, a highly recommended course for both React beginners and intermediate developers.
2. Meta Front-End Developer Professional Certificate [Coursera]
If you are looking for the best Coursera course for React.js then you will be pleased with this React basic course by Meta on this list. This is an awesome course for anyone who wants to become a full-stack developer with React.js as a frontend.
This course is offered by the Meta and is taught by frontend developers and React experts from Meta and this is also part of the popular Meta Frontend Developer certificate.
The “React Basics” course is also an integral part of various programs and provides a comprehensive introduction to React, a powerful JavaScript library for building user interfaces in web and mobile applications.
Delivered in English, the course, with 98,515 participants already enrolled, is designed to equip learners with fundamental skills for creating scalable, maintainable websites, and apps using React.
Taught by Meta Staff, the course covers key topics such as using reusable components, organizing React projects, passing data between components, creating dynamic and interactive web pages, and building applications in React.
With a 4.7 rating from 1,475 reviews, the course is accessible to beginners, requiring only basic internet navigation skills. Participants can earn a shareable certificate, add it to their LinkedIn profiles, and leverage the gained skills to boost their careers.
Here is the link to join this course — React basic course by Meta

The curriculum includes 21 quizzes, offering a flexible schedule for learners to progress at their own pace over approximately 26 hours. With hands-on projects and industry expert insights, the “React Basics” course is a valuable resource for those looking to embark on a career in mobile development.
Given Coursera’s popularity in bringing the best courses from the world’s top universities and top companies like IBM, Google, Meta, and AWS, I highly recommend this course to anyone who wants to learn React.js in 2025. More than 98K people have already joined this course and you can be the next.
By the way, if you find Coursera courses useful, which they are because they are created by reputed companies and universities around the world, I suggest you join Coursera Plus, a subscription plan from Coursera that gives you unlimited access to their most popular courses, specialization, professional certificate, and guided projects
3. Complete React Developer in 2025 (w/ Redux, Hooks, GraphQL)
This is one of the most advanced and complete courses to learn React.js in 2025. This course covers almost everything a React developer needs to know from context APIs to Hooks to Redux.
By joining this course you will master the React ecosystem from scratch and become a senior React developer, a top 10% React.js developer who knows React.js in depth including Hooks, Redux, GraphQL, and much more.
This course was created by famous instructor Andrei Neagoie, who is an awesome instructor and puts a lot of value in his courses with exercises, assessments, and projects.
You will build a massive E-commerce app with Redux, Hooks, GraphQL, Context API, Stripe, and Firebase in this course, step by step, and learn all these key skills along the way.
Here is the link to join this course — Complete React Developer in 2025 (w/ Redux, Hooks, GraphQL)
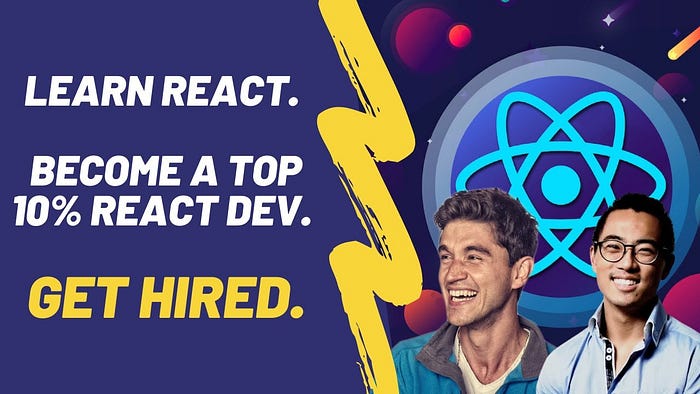
Btw, you would need a ZTM membership to watch this course which costs around $29 per month but also provides access to many super engaging and useful courses like this Python course and this JavaScript Web Projects: 20 Projects to Build Your Portfolio course. You can also use the FRIENDS10 coupon to get 10% OFF.
4. React — The Complete Guide (incl Hooks, React Router, Redux)
I
am a big fan of Maximilian Schwarzmüller and he is probably the best
instructor out there in Udemy or another online course website for Angular and React.js.
Having attended his courses like Angular — The Complete Guide and React Native — The Practical Guide,
I can say that they are simply awesome and he explains everything in
such a way that you will understand even complex concepts without making
a sweat.
On top of that, he is very hands-on and he builds his
applications and examples from scratch, explaining every single thing he
is doing, which makes it easy to follow instructors and learn along.
Like the previous React course, this one also covers all important React fundamentals like building components and leveraging them to build complex GUI. It also touches base on React for managing state and how to use Redux along with React JS.
Here is the link to join this React course — React — The Complete Guide

The course is also very comprehensive with more than 33.5 hours of material. You will start with fundamentals and then learn to code in React, followed up by testing and debugging your React application.
In short, a complete guide to learning React.js in 2025. Highly Recommended !!
5. Reintroducing React: V16 and Beyond [Educative]
Frontend frameworks are always changing, and React is no exception. While the fundamental principles have stayed the same, the way we write React applications has evolved over the last 12 months.
This course can teach you all the new features of React and also how to use React in a more modern way in an interactive way.
This course was created by Ohans Emmanuel, who has extensive experience with React and building interactive and scalable consumer-facing products.
You’ll start by exploring the new lifecycle methods, and then you’ll go on to learn about state management with the context API. You will also learn advanced topics like React memo, lazy loading, hooks, and more.
Here is the link to join this course — Reintroducing React: V16 and Beyond
Btw, Educative is a unique learning platform with its text-based, hands-on learning.
If you find Educative courses useful, which they are, particularly their Grokking courses like Grokking System Design Interview and Coding Patterns then consider getting an Educative subscription that provides access to all of their courses for just $14.9 per month. It’s a cost-effective and better way to learn on Educative.
6. React.js: Getting Started [Pluralsight course]
This is one of the best courses to learn about the React library on Pluralsight.
The instructor Samer Buna is a software developer, technologist, and
product delivery expert and has done a wonderful job explaining React
concepts to beginners.
This course covers all the features
offered by React JS and explains their advantages and disadvantages
relative to the other popular options available like Angular or Vue JS.
It
also discusses some of the important topics like React’s one-way
reactive data flow, the virtual DOM, and JSX syntax for describing
markup.
In short, one of the best courses to start learning React JS on Pluralsight, even for people who are relatively new to front-end programming and JavaScript.
Here is the link to join this course — React.js: Getting Started

Btw, you need a Pluralsight membership
to access this course. If you are already a member then just join this
course but if you are not then you can become a Pluralsight member by
option for a monthly subscription of $29 a month or a yearly
subscription of $299 per year.
This gives you access to more
than 5000+ high-quality courses to learn the latest technologies. If you
don’t want to commit, you can also try this course by signing up for
the 10-day free trial on Pluralsight which provides you 200 minutes of access to all its courses, including this one.
7. Advanced React and Redux: 2025 Edition
This
is another awesome course from Stephen Grider on React and Redux. In
this course, Stephen provides detailed walkthroughs on advanced React
and Redux concepts like Authentication, Testing, Middlewares, HOCs, and
Deployment.
You will learn how to build a scalable API with authentication using Express, Mongo, and Passport and learn the differences between cookie-based and token-based authentication.
You
will also figure out what a Higher Order Component is and how to use it
to write dramatically less code and learn to set up your testing
environment with Mocha and Chai.
In short, an advanced-level
React and Redux courses that you can use along with the first React
course mentioned in this article to become a complete React developer.
Here is the link to join this course — Advanced React and Redux: 2025 Edition

8. FullStack React [Book]
If you like reading books along with watching the course then this React book is for you. A wise developer will want to have a well-rounded understanding of web development. To acquire this, you should employ a few of the other disciplines utilized by seasoned professionals.
In this book, you will learn Full Stack React from experts like Anthony Accomazzo, Nate Murray, Ari Lerner, Clay Allsopp, David Guttman, and Tyler McGinnis. There is hardly any book where you get a chance to learn from so many experts in one place.
This book broadens our understanding of multiple frameworks and ways to employ full-stack development. You will be a confident developer, after digesting the topics in this important text.
All contributors are respected authors and developers, with extensive experience with many languages and frameworks
Here is the link to buy this book —. FullStack React

And, if you need more choices, you can also check out this list of best React.js books to learn in 2025.
9. React Developer course from AlterClass
This is another amazing React course that I liked. Created by @Greg D’Angelo, a senior software engineer and mentor specializing in teaching React, JavaScript and much more this course will teach you all the essential concepts a React Developer should learn.
Here are the main concepts you will learn in this course:
- React Fundamentals
- React State Management
- React Styling
- React Hooks
- React Routing
- React Testing
- Firebase Auth & Database
- Deployment and Hosting
You can join this course to master React and build real-world applications. Also worth noting is that the first module of this course, React Fundamentals is free and worth joining.
Here is the link to join this course — React Developer course from AlterClass
10. The Complete React Web Developer Course (with Redux) [Udemy]
This
is another awesome course on Udemy to learn how to build and launch
React web applications using React v16, Redux, Webpack, React-Router v4,
and related technology.
The Complete React Web Developer Course 2
starts with the React fundamentals like components, JSX, etc, and
covers everything you’ll need to build and launch React to web apps.
You’ll
learn what it takes to build and launch a React app, from the first
line of code to the final production deployment. yes, the course is
truly hands-on and you will learn to React not by watching but by doing.
It also has programming challenges and exercises
which are designed to test and reinforce whatever you have learned. You
will also build two real-world React web apps Indecision, a
decision-making app, and an expense manager called Budget.
Here is the link to join this course — The Complete React Web Developer Course (with Redux)
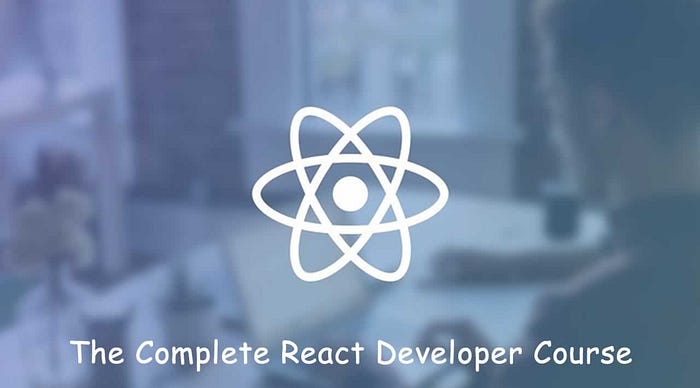
These
apps have all the features you’d expect from a real-world application
including authentication, user accounts, routing, testing, form
validation, database storage, and more. These development exercises will
provide you with valuable experience, which you need to clear any React
interview.
The author Andrew Mead is a full-stack developer
and a great teacher who has in-depth knowledge of the subject he
teaches. This course is truly awesome and hands-on and I highly
recommend this to all programmer who wants to become professional web
developers using React.
This
course is designed around one goal: turning you into a professional
React developer capable of developing, testing, and deploying real-world
production applications and it has lived up to its promise.
11. Complete Intro to React, v8 on Frontend Masters
This is another impressive and most up-to-date online course for developers to build real-world applications using the modern React framework (version 18.x+).
Led by the experienced instructor Brian Holt from Stripe, this Frontend Masters course provides a comprehensive introduction to React, equipping learners with the knowledge and skills to leverage the latest features and tools in their projects.
From the very beginning, you will be guided through a step-by-step journey, starting with the React fundamentals and gradually progressing to advanced concepts. The course covers all essential topics such as React hooks, effects, context, and portals, enabling developers to harness the full power of React in their applications.
Along the way, you will also get chance to work with various tools from the React ecosystem, including Vite, ESLint, Tailwind CSS, React Router, and React Query.
By incorporating these tools, you will gain a holistic understanding of how different components can be combined to create a complete application using the React library.
The best thing about the course is that it's hands-on and it also has a project where you will build an application for browsing adoptable pets, providing a practical context for applying the concepts learned.
Here is the link to join this course — Complete Intro to React

On top of that, the production quality of the course is top-notch, with clear audio, well-designed slides, and high-definition video, enhancing the overall learning experience, you won’t find that in any other place.
The course materials, including code samples and additional resources, are easily accessible, enabling learners to reinforce their understanding at their own pace.
12. React 18 Tutorial and Projects Course — 2025 Edition
This is a new React.js course I have found and it's perfect for anyone who wants to learn React.js in 2025, especially by doing projects.
This project is created by popular Udemy instructor John Smilga and it’s a hands-on course to learn React 18 in depth.
The course not only teaches you React.js but also Axios, Router 6, Query 5, Redux Toolkit, and much more. And the best thing about the course is that you will build 25 projects on this course which is amazing.
Projects include all kinds of projects like an e-commerce project, HackerNews clone, Github users, Color Generator, Unsplash image project, and much more. This is also a massive course with more than 77 hours of content and more than 22 articles
Here is the link to join this project — React 18 Tutorial and Projects Course — 2025 Edition

That’s all about the best Udemy, Coursera and Educative courses to learn React and Redux frameworks for modern web development in 2025. The list not only includes online courses to learn React.js but also interactive, coding-based courses as well React certificates, books, and project-based React courses as well.
If
you are looking to build your website or looking for a job in the web
development space, these online React.js courses will help you a lot.
Other Programming and Web Development Courses you may like
The 2025 Web Developer RoadMap
5 Free Spring Framework Courses for Java Developers
Top 5 Courses to Learn Web Development in 2025
5 Courses to Learn Big Data and Apache Spark
Top 10 JavaScript Tutorials and Courses for Web Developers
Top 5 Courses to Learn Spring Boot in 2025
Best Apache Kafka Courses — 2025
5 Courses to Learn to React Native in 2025
Top 5 Courses to Learn TypeScript for Web Developers
The 2025 React JS Developer RoadMap
10 Free Courses to learn Reactjs, Context API, and Hooks
6 Best Places to Learn React.js in 2025
10 React Projects Ideas for Beginners in 2025
Thanks for reading this article so far. If you like these online React and Redux courses then please share them with your friends and colleagues. If you have any questions or feedback then please drop a note.
P. S. — If you are looking for some free courses to start your React.js journey then you can also check out this list of Free React.js Courses for beginners and Web developers.