Java coding interview questions answers
Java coding interview questions are mostly some programming exercises which is asked to be solved in the java programming language in a limited time during any core Java or J2EE interview. No matter whether you have 2 years of experience or 4 years of experience, there is always some coding interview question in the Java programming job you are applying for. Actually, questions related to Java Coding are increasingly getting popular in Java interviews for two reasons, first its difficult to crack Java coding interview questions than answering fact-based questions like Why String is immutable in Java or Why main is static in Java etc.
Java coding interview questions are mostly some programming exercises which is asked to be solved in the java programming language in a limited time during any core Java or J2EE interview. No matter whether you have 2 years of experience or 4 years of experience, there is always some coding interview question in the Java programming job you are applying for. Actually, questions related to Java Coding are increasingly getting popular in Java interviews for two reasons, first its difficult to crack Java coding interview questions than answering fact-based questions like Why String is immutable in Java or Why main is static in Java etc.
Second reason of popularity of Coding question in Java interviews is read need of good developers who are required to do a lot of coding in projects rather than doing some maintenance works.
Since Java is front line language for any server-side application, and as the complexity of the business process and need of performance is increasing, its obvious that the amount of coding skill required in Java programmers are getting increased with every passing day, which effectively means Java coding questions are top of any list of core Java interview question.
These Coding interview questions are collected from various Java programming interviews, from friends and colleagues, and can be a good starting point to refresh your coding skills before appearing on any Java interviews. These basics Java Programs and logical questions can also be a good resource for learning to program and improving your problem-solving skills in Java.
Anyway, you may be able to solve and find answers of these Java coding questions by yourself, but if you stuck do a google, and you can get many alternative ways to solve these problems.
Since Java is front line language for any server-side application, and as the complexity of the business process and need of performance is increasing, its obvious that the amount of coding skill required in Java programmers are getting increased with every passing day, which effectively means Java coding questions are top of any list of core Java interview question.
These Coding interview questions are collected from various Java programming interviews, from friends and colleagues, and can be a good starting point to refresh your coding skills before appearing on any Java interviews. These basics Java Programs and logical questions can also be a good resource for learning to program and improving your problem-solving skills in Java.
16 Programming Assignments, Homework, and Coding Interview questions answers in Java
Here are my list of 10 Java coding interview questions and answers, which is good to prepare before appearing on any Java interviews. As I said Java coding questions are mostly based on programming, logical analysis and problem-solving skill and are on top of any list of tough Java interview questions, so better to get it right in first place.Anyway, you may be able to solve and find answers of these Java coding questions by yourself, but if you stuck do a google, and you can get many alternative ways to solve these problems.
Sometimes knowing more than one way to solve any programming question or coding problem in Java also helps to impress the interviewer. This list mainly contains basic programs asked on Interviews.
1. Write a Java program to replace certain characters from String like
public String replace(String str, char ch)
This is a tricky Java coding interview question and it was asked in one of the written test my friend had appeared recently.
This Java coding question can be solved in multiple way like by using charAt() or substring() method, but any approach throws couple of follow-up question like you may be asked to write two version to solve this coding exercise, one by using recursion and other by using Iteration.
They may also ask you to write JUnit test for this function which means handling null, empty string etc. By the way this programming question is quite common on technical interviews not just Java but also C, C++ or Scala, but knowing API definitely helps to produce better solution quickly.
They may also ask you to write JUnit test for this function which means handling null, empty string etc. By the way this programming question is quite common on technical interviews not just Java but also C, C++ or Scala, but knowing API definitely helps to produce better solution quickly.
2. Write a Java program to print different kind of patterns like Triangular pattern, box pattern, Pyramid patter of stars, numbers or alphabets.
This is one of the popular Java homework assignment as well as popular program to learn programming and coding.
The trick to solve all pattern questions are using loops. You need to know how to nest loops into each other as well as how to use continue and break to come out from inner and outer loop.
You also need to understand how to use print() and println() methods to print characters in same line and different lines. In the past, I have showed you how to print left and right triangular pattern and pyramid pattern of stars as well as pyramid pattern of alphabets.
Here are a couple of patterns you can try printing to improve your coding skill in Java.
3. Write a Java program to print Fibonacci series up to 100?
This is one of the most popular coding interview questions asked in Java programming language. Even though, a Writing program for Fibonacci series is one of the basic Java program, not every Java developer get it right in interview.
Again interview can ask to solve this programming interview question, by using recursion or Iteration. This Java programming question also test your problem-solving skills and if you come up with an original solution, that may even help. See here for complete code example of Fibonacci series in Java
4. FizzBuzz problem : Write a Java program that prints the numbers from 1 to 50. But for multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". For numbers which are multiples of both three and five print "FizzBuzz"
This is also one of the classical programming questions, which is asked on any Java programming or technical interviews. This question is very basic but can be very trick for programmers, who can't code, that's why it is used to differentiate programmers who can do coding and who can't.
Here is a sample Java program to solve the FizzBuzz problem :
You can also see this post to solve FizzBuzz in Java 8 using functional programing, lambda and stream.
5. Write a Comparator in Java to compare two employees based upon there name, departments, and age?
This is a pure Java based Coding exercise. In order to solve this Java coding or programming interview question, you need to know What is a Comparator in Java and how to use compare method in Java for sorting Object. Sorting is one of the most logical and practical question on technical interview and ability to sort Java object is must to code in Java.
This article helps you to solve this Java coding question by explaining how to sort object in Java using Comparable and Comparator. Just remember that Comparable has compareTo() method and use to sort object based upon there natural order e.g. numeric order for number, and alphabetic order for String, while Comparator can define any arbitrary sorting.
A good follow-up question can also be the difference between Comparator and Comparable in Java, so be ready for that. You can also see these Java Interview Courses for better preparation, it includes all important topics and questions to quickly prepare for your next Java interview.
6. Design a vending machine in Java which vends Item based upon four denomination of coins and return coin if there is no Item. (solution)
This kind of Java coding interview question appears in written test and I believe if you get it right, you are almost through the Interview. These kinds of problem-solving questions in Java are not easy, you need to design, develop and write JUnit test within 2 to 3 hours and only good Java developers, with practical coding experience, can solve this kind of Java programming question.
4. FizzBuzz problem : Write a Java program that prints the numbers from 1 to 50. But for multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". For numbers which are multiples of both three and five print "FizzBuzz"
This is also one of the classical programming questions, which is asked on any Java programming or technical interviews. This question is very basic but can be very trick for programmers, who can't code, that's why it is used to differentiate programmers who can do coding and who can't.
Here is a sample Java program to solve the FizzBuzz problem :
public class FizzBuzzTest{ public static void main(String args[]){ for(int i = 1; i <= 50; i++) { if(i % (3*5) == 0) System.out.println("FizzBuzz"); else if(i % 5 == 0) System.out.println("Buzz"); else if(i % 3 == 0) System.out.println("Fizz"); else System.out.println(i); } } }
5. Write a Comparator in Java to compare two employees based upon there name, departments, and age?
This is a pure Java based Coding exercise. In order to solve this Java coding or programming interview question, you need to know What is a Comparator in Java and how to use compare method in Java for sorting Object. Sorting is one of the most logical and practical question on technical interview and ability to sort Java object is must to code in Java.
This article helps you to solve this Java coding question by explaining how to sort object in Java using Comparable and Comparator. Just remember that Comparable has compareTo() method and use to sort object based upon there natural order e.g. numeric order for number, and alphabetic order for String, while Comparator can define any arbitrary sorting.
A good follow-up question can also be the difference between Comparator and Comparable in Java, so be ready for that. You can also see these Java Interview Courses for better preparation, it includes all important topics and questions to quickly prepare for your next Java interview.
6. Design a vending machine in Java which vends Item based upon four denomination of coins and return coin if there is no Item. (solution)
This kind of Java coding interview question appears in written test and I believe if you get it right, you are almost through the Interview. These kinds of problem-solving questions in Java are not easy, you need to design, develop and write JUnit test within 2 to 3 hours and only good Java developers, with practical coding experience, can solve this kind of Java programming question.
What helps you is to keep practicing your coding skill even before interview.
See this programming exercise in Java to get yourself going. I personally like to ask programming questions, which test your object oriented design skills like designing ATM machine, designing parking lot or implementing logic for Traffic Signal controller.
See this programming exercise in Java to get yourself going. I personally like to ask programming questions, which test your object oriented design skills like designing ATM machine, designing parking lot or implementing logic for Traffic Signal controller.
7. Write a Java program to check if a number is Armstrong or not ?
Another popular logical coding interview questions in Java, which is based on programming logic. In order to answer this programming question, you need to know what is Armstrong number, but that is not a problem because question may specify that and even provide sample input and output.
The key thing to demonstrate is logic to check if a number is Armstrong or not. In most cases, you can not use utility methods defined by logic and you need to produce logic by yourself by using basic operators and methods.
By the way, this is also one of the basic programming questions and I have already provided a solution for this. I suggest seeing this Java program to find Armstrong Number in Java to answer this coding question
8. Write a Java program to prevent deadlock in Java?
Some of the programming or coding interview question is always based on a fundamental feature of Java programming language like multi-threading, synchronization, etc.
Since writing deadlock proof code is important for a Java developer, programming questions which requires knowledge of concurrency constructs becomes popular coding question asked in Java Interviews.
The deadlock happens if four conditions is true like mutual exclusion, no waiting, circular wait and no preemption. If you can break any of this condition than you can create Java programs, which are deadlock-proof.
One easy way to avoid deadlock is by imposing an ordering on acquisition and release of locks. You can further check How to fix deadlock in Java to answer this Java programming questions with coding in Java
The deadlock happens if four conditions is true like mutual exclusion, no waiting, circular wait and no preemption. If you can break any of this condition than you can create Java programs, which are deadlock-proof.
One easy way to avoid deadlock is by imposing an ordering on acquisition and release of locks. You can further check How to fix deadlock in Java to answer this Java programming questions with coding in Java
9. Write Java program to reverse String in Java without using API functions?
Another classic Java programming or coding exercise mostly asked on 2 to 5 years experienced Java interviews. Despite being simple answering this coding question is not easy, specially if you are not coding frequently. Its best to prepare this programming question in advance to avoid any embarrassment during interviews. I suggest to see this post which shows How to reverse String using recursion in Java
10. Write a Java program to find if a number is a prime number or not? (solution)
One more basic Java program, which made it's way to Interviews. One of the simplest coding question and also a very popular Java programming exercise. The beauty of these kinds of logical questions is that, they can really test the basic programming skills or a coder, programmer or developer.
Not just problem solving, you can also check there coding style and thought process.
By the way. you can also check the Java Programming Interview Exposed for many of such Java coding interview questions. It also covers other important topics e.g. Spring, Hibernate, Java Fundamentals, Object-oriented programming etc.
11. How to Swap two numbers without using a third variable in Java?
This Java program might require just four lines to code, but it's worth preparing. Most of the programmers make the same kind of mistakes while writing solutions for this program like Integer overflow, they tend to forget that an integer can overflow if its limit is exceeded, which is not very big. Sure shot way to answer these programming questions is to use the XOR trick to swap numbers, as mentioned in that blog post.
12. Create a Java program to find the middle node of the linked list in Java in one pass?
Any list of programming questions is incomplete without any questions from a linked list, arrays, and string, these three forms bulk of coding questions asked on Java interviews. The trick to solving this problem is to remember that the last node of the linked list points to null and you can trade memory with speed.
Sometimes your approach to come to a two-pointer solution really matters, by taking rational steps as mentioned above, you can sound more intelligent, a problem solver, and genuine. A quick solution to this programming question can be found here.
13. How to find if a linked list contains a cycle or not in Java? (solution)
Another programming question is based on a linked list. By the way, this coding question is a bit tricky than the previous one, but this can also be solved using two pointer approach. If the linked list has a cycle, then the fast pointer will either catch the slow pointer or point to null.
11. How to Swap two numbers without using a third variable in Java?
This Java program might require just four lines to code, but it's worth preparing. Most of the programmers make the same kind of mistakes while writing solutions for this program like Integer overflow, they tend to forget that an integer can overflow if its limit is exceeded, which is not very big. Sure shot way to answer these programming questions is to use the XOR trick to swap numbers, as mentioned in that blog post.
12. Create a Java program to find the middle node of the linked list in Java in one pass?
Any list of programming questions is incomplete without any questions from a linked list, arrays, and string, these three forms bulk of coding questions asked on Java interviews. The trick to solving this problem is to remember that the last node of the linked list points to null and you can trade memory with speed.
Sometimes your approach to come to a two-pointer solution really matters, by taking rational steps as mentioned above, you can sound more intelligent, a problem solver, and genuine. A quick solution to this programming question can be found here.
13. How to find if a linked list contains a cycle or not in Java? (solution)
Another programming question is based on a linked list. By the way, this coding question is a bit tricky than the previous one, but this can also be solved using two pointer approach. If the linked list has a cycle, then the fast pointer will either catch the slow pointer or point to null.
If you want to practice more such questions based upon the linked list, array, and String and asked in various companies before, then I suggest you check the Cracking the Coding Interview, it contains more than 190 questions from various tech companies, startups, and service-based companies like Infosys, TCS, and Wipro in India.
14. Implement Producer Consumer design Pattern in Java using wait, notify, and notifyAll method in Java?
Similar to deadlock-related programming interview questions, this is also used to test programmers' ability to write bug-free concurrent programs in Java. These coding questions can be difficult if you haven't used wait and notify before, you can confuse yourself as hell on different aspects like which condition to check, on which lock you should be synchronized, etc. I suggest following here to answer this multithreading-based programming interview question.
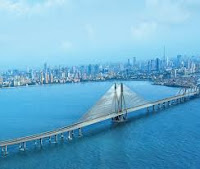
15. Write a Java program to calculate the Factorial of a number in Java?
This Java coding interview question is also based on a list of basic Java programs for beginners. As usual, you better remember how to calculate factorial and how to code solutions using loop and recursive method calls. For a complete code solution of this programming question, see the Java program to calculate factorial
16. How to design a Trade Position Aggregator or Portfolio Management Software in Java?
14. Implement Producer Consumer design Pattern in Java using wait, notify, and notifyAll method in Java?
Similar to deadlock-related programming interview questions, this is also used to test programmers' ability to write bug-free concurrent programs in Java. These coding questions can be difficult if you haven't used wait and notify before, you can confuse yourself as hell on different aspects like which condition to check, on which lock you should be synchronized, etc. I suggest following here to answer this multithreading-based programming interview question.
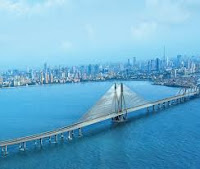
15. Write a Java program to calculate the Factorial of a number in Java?
This Java coding interview question is also based on a list of basic Java programs for beginners. As usual, you better remember how to calculate factorial and how to code solutions using loop and recursive method calls. For a complete code solution of this programming question, see the Java program to calculate factorial
16. How to design a Trade Position Aggregator or Portfolio Management Software in Java?
This is another interesting object oriented design problem which is asked to Java programs. In this program you need to create a core Java application which can calculate trade position in real time.
Live trades will be fed to system, both buy and sell and it needs to update the position on real time. Position means how many shares of a particular company a particular account is holding. You need to support both multiple accounts and multiple trading symbol.
You can try this problem yourself but if you stuck you can see my solution to create Trade aggregator in Java for a step by step guidance.
These are some of the Java coding interview questions and answers, which appear frequently on Java Programming interviews. I have included links, with some of my blog posts, which discusses answers of these Java coding question, but you can also find answers by doing google yourself. Please share what kind of Programming, logical, Problem solving or coding-related questions, asked to you in Java interviews?
Other Java and Coding Interview questions from Java67 blog
- 10 tricky Java interview questions - Answered
- 189 Coding Interview Questions from Amazon, Google, and Microsoft
- 10 Linux and UNIX interview questions - Answered
- Best Java Collection interview questions answers for practice
- Top 40 Java Telephonic Interview Questions
- Top 20 Hibernate Interview Questions with Answers
- Top 21 Spring MVC Interview Questions
- Top 10 RESTful Web Services Interview Questions
- Top 30 Java OOP Interview Questions with Answers
- 10 Java Developer Interview Courses to Prepare Next Interview
Thanks for reading this article so far. If you like these Java Programming Coding Interview Questions then please share them with your friends and colleagues. If you have any questions or suggestions then please drop a note.
Good collection of Coding and programming question in Java. Surprised not to see Generics, Data structure's, LinkedList etc
ReplyDeleteHere is my list of Java programming interview question :
ReplyDeleteWrite a Java program to create Thread and Stop Thread (remember there is no stop method for stopping thread in Java)
Write a Java program to sort Map based on keys without using TreeMap
Write a Java program to create deadlock ? how do you fix it ?
Write a Java program to find maximum number in Array
I agree that these Java programming question are basic but in my opinion you need to be better than average programmer to be able to write code which does that correctly.
How to use the java program to basic
DeleteBasic concept ask to me plz
Deletenew TreeSet(map.keySet());
DeleteIt's probably not the desired answer, but it is a one-line solution that answers the question as asked and demonstrates knowledge of data structures.
how to star print in rotate style..
Deletevery nice collection of information and improve them.
ReplyDeleteRe question "Write a Java program to replace certain characters from String" seems like the example method is missing a replaceWith parameter no?
ReplyDeletepublic String replace(String str, char old, char new)
I agree...
DeleteWrite a Java program if 1st digit is 1 then it is replace by 0 and vise vera, and for 2nd digit if 1st and 2nd digit are 1 make it 0 and vise versa in an input array.
DeleteAuthor said it was a tricky question. Maybe the trick is realizing it is incorrectly specified :D
Deletethanks for the questions...they really helped....plus your blog is awesome for an aspiring programmer like me..keep it up...:)
ReplyDeleteThis is the coding question asked to me in couple of interviews :
ReplyDelete1) How to remove duplicates from an array of Integers, without using API methods in Java?
2) Code to implement binary search in Java?
let me know, if anyone has answers for these questions?
This is the basic function for removing the duplicates from the array
Deletepublic class Array {
public static void main(String args[]){
int count = 0 ;
int [] A = new int[] {1,2,3,4,4,4,4,4,5,5,5,6,5,5,5,5,5,8};
int c = A.length;
System.out.println(c);
System.out.println("Array starts");
for (int i = 0 ; i< c ; i++){
for (int j = i+1 ; j < c ; j++) {
if (A[i] == A[j]) {
for (int p = j; p < c - 1; p++) {
A[p] = A[p + 1];
}
j= j -1;
c = c - 1;
}
}
}
for (int i = 0 ; i < c; i++){
System.out.println(A[i]);
}
public static void main(String[] args)throws Exception {
Delete// TODO code application logic here
Scanner in =new Scanner(System.in);
int n=in.nextInt();
int num[]=new int[n];
for(int i=0;i<n;i++)
num[i]=in.nextInt();
String result="";
boolean b=true;
for(int i=0;i<n-1;i++)
{
for(int j=i+1;j<n;j++)
if(num[i]!=num[j])
b=true;
else
{
b=false;
break;
}
if(b==true)
System.out.print(num[i]);
}
System.out.print(num[n-1]);
}
Some of these questions have appeared in a written test on Java programming, I had given few months back. I would personally like to see more questions from class loading involving static and non static variables, questions which are confusing enough on overloading and overriding, questions which has some tricky concept on it etc. Though these are still good for beginners, you would like to add few more for experienced Java programmers.
ReplyDeleteThis was a coding question which i was asked
ReplyDeleteWrite a program, to find a missing number in array of 100 numbers
apply the formula sum of n numbers n * (n+1) /2
Deletesuppose number 8 is missing from the array 1..10.
Sum of 10 numbers : 10* 11/2 = 55
Sum of numbers from array = 47
missing number = 55-47 =8
how can u give that sum of 10 numbers where we dont know which number is missing among the 100 numbers?
DeleteJust Subtract the current number from previous number. If the difference is 2, (Previous number + 1) is the missing number.
Deletethis will only work if array is sorted but its not stated in the question...
DeleteWrite a program in java to change number into words
ReplyDeletefor example 2341 into Two Thousand Three Hundred Fourthy One
Hey Ujjwal,
DeleteThis program should work for any four digit whole number.
Cheers.
import java.util.Scanner;
public class Num2Txt {
private static final String[] tensNames = {
"",
" Ten",
" Twenty",
" Thirty",
" Forty",
" Fifty",
" Sixty",
" Seventy",
" Eighty",
" Ninety"
};
private static final String[] numNames = {
"",
" One",
" Two",
" Three",
" Four",
" Five",
" Six",
" Seven",
" Eight",
" Nine",
" Ten",
" Eleven",
" Twelve",
" Thirteen",
" Fourteen",
" Fifteen",
" Sixteen",
" Seventeen",
" Eighteen",
" Nineteen"
};
public static void main(String []args)
{
Scanner reader = new Scanner(System.in);
System.out.println("Enter a whole number (max 4 digits): ");
int n = reader.nextInt();
System.out.println(n);
reader.close();
int units = n%10;
int tens = (n%100 - units)/10;
int hundreds = (n%1000 - (tens+units))/100;
int thousands = (n-(n%1000))/1000;
System.out.println("In the thousands place: " +thousands);
System.out.println("In the hundreds place: " +hundreds);
System.out.println("In the tens place: " +tens);
System.out.println("In the units place: " +units);
if(n==0)
System.out.println("Zero");
else if(n<10)
System.out.println(numNames[units]);
else if(n<100)
System.out.println(tensNames[tens]+""+numNames[units]);
else if(n<1000)
{
if(tens==0&&units==0)
System.out.println(numNames[hundreds]+" Hundred");
else if(tens==0&&units!=0)
System.out.println(numNames[hundreds]+" Hundred and"+numNames[units]);
else
System.out.println(numNames[hundreds]+" Hundred and"+tensNames[tens]+""+numNames[units]);
}
else if(n<10000)
{
if(hundreds==0&&tens==0&&units==0)
System.out.println(numNames[thousands]+" Thousand");
else if(hundreds==0&&tens==0&&units!=0)
System.out.println(numNames[thousands]+" Thousand and"+numNames[units]);
else if(hundreds==0&&tens!=0)
System.out.println(numNames[thousands]+" Thousand and"+tensNames[tens]+""+numNames[units]);
else if(hundreds!=0&&tens==0&&units==0)
System.out.println(numNames[thousands]+" Thousand"+numNames[hundreds]+" Hundred");
else
System.out.println(numNames[thousands]+" Thousand"+numNames[hundreds]+" Hundred and"+tensNames[tens]+""+numNames[units]);
}
else System.out.println("Please enter a four digit whole number!(0-9999)");
}
}
Find the count of number in list? ex: 1,1,1,1,2,2,3,3,4,4,4,4,4
ReplyDeleteo/p: 1-4,2-2,3-2,4-5
count sort/hashmap
DeleteWrite a program to print all the permutations of a string (eg: if abc is a string then o/p should be as abc,acb,bac,bca,cab,cba. i.e 3 factorial ways.) this an interview question given out of 200 - < 5 persons solved this
ReplyDeleteWell I answered it so I might as well post it here..
Delete(Might be some better String/StringBuilder API functions than the ones I used)..
public static List permutations(String str) {
List res = new ArrayList();
if (str.length() < 2) {
res.add(str);
return res;
}
List currRes = null;
for (int i=0; i<str.length(); i++) {
StringBuilder newStr = new StringBuilder(str);
char tmpchar = newStr.charAt(i);
newStr.setCharAt(i, newStr.charAt(0));
newStr.setCharAt(0,tmpchar);
currRes = permutations(newStr.substring(1));
for (String s : currRes) {
res.add(newStr.charAt(0) + s);
}
}
return res;
}
Here is what I did :
Deletestatic String insertCharAtThisPosition( String str, char c, int position){
if ( str == null ) return "";
if ( position == 0 ) return c + str;
if ( position >= str.length() ) return str + c;
return str.substring(0,position) + c + str.substring(position);
}
static List permutations ( String str){
List r = new ArrayList();
if ( str == null || str.length() == 0 ) return r;
if ( str.length() == 1 ){ r.add(str); return r; }
List permuationsSmaller = permutations(str.substring(1));
for ( int i = 0 ; i < str.length() ; i++ ){
for ( String permutation : permuationsSmaller ){
r.add( insertCharAtThisPosition(permutation, str.charAt(0),i) );
}
}
return r;
}
well this is what i could come up with
Deletepublic class Algorithm {
public char tmp ,tmp2;
public void generate(int n , StringBuilder str){
if (n==1){
System.out.println(str);
}
else {
for (int i = 0 ; i < n-1 ;i++){
generate(n-1 ,str);
if (n%2==0){
tmp = str.charAt(i);
tmp2 = str.charAt(n-1);
str.setCharAt(i,tmp2);
str.setCharAt(n-1,tmp) ;
}
else{
tmp = str.charAt(0);
tmp2 = str.charAt(n-1);
str.setCharAt(0,tmp2);
str.setCharAt(n-1,tmp);
}
}
generate(n-1,str);
}
}
public static void main(String [] args ){
Algorithm a = new Algorithm();
// int [] A = new int [] {1,2,3,4} ;\
Scanner sc = new Scanner(System.in);
System.out.println("Enter string");
String str = sc.nextLine();
StringBuilder s = new StringBuilder(str);
a.generate(s.length(),s);
}
3. Write a program that reads from the user four integers representing the numerators and denominators of two fractions, calculates the results of the two fractions and displays the values of the fractions sum, subtraction, multiplication and division.
ReplyDeleteSample run:
Enter the numerator and denominator of the first fraction: 6 4
Enter the numerator and denominator of the second fraction: 8 5
The sum is: 3.1
The subtraction is: -0.1
The multiplication is: 2.4
The division is: 0.9375
Maybe?
Deleteimport java.util.Scanner;
public class FractionCalculations {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner reader = new Scanner(System.in);
System.out.println("Enter first number numerator: ");
int num1 = reader.nextInt();
System.out.println("Enter first number denominator: ");
int den1 = reader.nextInt();
System.out.println("Enter second number numerator: ");
int num2 = reader.nextInt();
System.out.println("Enter second number denominator: ");
int den2 = reader.nextInt();
reader.close();
//IMPORTANT: CAST NUMERATOR TO DOUBLE
double number1 = (double)num1/den1;
double number2 = (double)num2/den2;
System.out.println("Sum: "+(number1+number2));
System.out.println("Subtraction: "+(number1-number2));
System.out.println("Multiplication: "+(number1*number2));
System.out.println("Division: "+(number1/number2));
}
}
2. Write a program that reads in from the user an integer (num) between 1000 and 9999. Then it prompts the user to enter an integer (d) between 0 and 9 and a character (ch). Your program should replace the second and the last digit in num with d and it should display the character that precedes (ch) followed by the number after the change and then the character that comes after (ch). Use the division and modulus operators to extract the digits from num.
ReplyDeleteSample run:
Enter an integer between 1000 and 9999: 2134
Enter a digit (between 0 and 9): 6
Enter a character: b
Number was 2134. Result: a2636c.
package com.Vikram.Program;
Deleteimport java.util.Scanner;
public class Prog
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.println("Enter Integer bertween 1000 - 9999 : ");
int i = sc.nextInt();
System.out.println("Enter a number between 0-9 :");
int j= sc.nextInt();
System.out.println("Enter a character :");
String c = sc.next();
char cc =c.charAt(0);
int cd1=cc;
cc++;
char cc7=cc;
cc--;
cc--;
char cc4=cc;
String k=i+"";
k=k.replaceFirst(k.charAt(1)+"",j+"");
String k1=k.substring(0,k.length()-1);
k1+=j;
k1=cc4+k1;
k1+=cc7;
System.out.println(k1);
}
}
In your Program
DeleteThis test case not satisfy
Enter Integer bertween 1000 - 9999 :
1123
Enter a number between 0-9 :
6
Enter a character :
b
out put:-a6126c
according to me o/p should be a1626c
below is I am giving solution check it is satisfy all your requirement:
static void replacingNumber(Integer num,int repNum, int prepost){
String str=String.valueOf(num);
int num2=prepost;
StringBuilder str2=new StringBuilder();
str2.append((char)--num2);
System.out.println(str2);
for(int i=0;i<str.length();i++){
if(i==1 || i==str.length()-1){
str2.append(repNum);
}else{
str2.append(str.charAt(i));
}
}
num2=prepost;
str2.append((char)++num2);
System.out.println(str2);
}
Maybe?
Deleteimport java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class StringTransformations {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner reader = new Scanner(System.in);
System.out.println("Enter number between 1000 and 9999: ");
int num = reader.nextInt();
System.out.println("Enter a digit between 0 and 9: ");
int dig = reader.nextInt();
System.out.println("Enter a character: ");
char c = reader.next().charAt(0);
reader.close();
List list = new ArrayList();
char[]temp = Integer.toString(num).toCharArray();
for(int i = 0; i < temp.length; i++)
{
list.add(temp[i]);
}
list.add(1, Integer.toString(dig).charAt(0));
list.set(list.size()-1, Integer.toString(dig).charAt(0));
int previousChar = c-1;
int nextChar = c+1;
list.add(0, (char) previousChar);
list.add((char) nextChar);
System.out.println(list.toString());
}
}
1. Write a program that takes three double values x0, v0, and t from the user and prints the value x0 +v0t +g t2/2, where g is the constant 9.78033. This value is the displacement in meters after t seconds when an object is thrown straight up from initial position x0 at velocity v0 meters per second.
ReplyDeleteSample run:
Enter the value of x0, v0 and t: 0 2 2
The displacement in meters after 2 seconds when an object is thrown straight up from initial position 0 at velocity 2meters per second is: 23.56066
such a easy question just use the basics of kinematics in your program.........AND IMPROVE YOUR PROGRAMMING SKILLS....
ReplyDeleteI think these questions are good to start with problem solving and algorithm building only, I wouldn't say they are challenging for a Java developer with just 1 year of experience. There is no algorithmic question from binary tree, B tree, balancing tree, traversal, linked list, stack, queue, graph, just bunch of practice questions.
ReplyDeleteWrite a program to print each line of a file that contains a particular pattern or string oa characters.The output should begin with the information on the word and character where this was found(x.y meaning x th word in the line and y thcharacter in the word)
ReplyDeleteE.g:
'ould'
Input: file.txt
How could tihs happen?
Re-mould the nearest output.
would this always happen?
Output:
2.1:How could tihs happen?
1.4:Re-mould the nearest output.
1.1:would this always happen?
Below is my code for this problem.
DeleteI am giving function
static void findPattern(String str,String pattern){
String str2[]=str.split("\\s");
Pattern p=Pattern.compile(pattern);
Matcher match=null;
int spaceCount=1;
int count=0;
for(int i=0;i<str2.length;i++){
match=p.matcher(str2[i]);
if(match.find()){
count=match.start();
spaceCount=i;
}
}
spaceCount++;
/*while(match.find()){
count=match.start();
}*/
System.out.println(spaceCount+"."+count+" : "+str);
}
About developing JUnit Tests...do they allow use of Eclipse or must be done from command line????
ReplyDeleteHi Admin,
ReplyDeleteExcellent and frequently ask list of java code interview questions with answers/ solutions. I am looking for the list of java questions and answers. While searching on google, I found this blog post. Thanks , It will surely help me. I partially read some of your blog and bookmark it for future use.
Hi,
ReplyDeleteThe following two questions were asked to me for a programing test:
1. Write a program to calculate the angle between the hour hand and the minute hand of a clock if the time is given in a string format. For example if the time is given as 12:15 the output should be 90degrees.
2. Write a program to evaluate a mathematical expression given in string format without using inbuilt eval() function. For example input would be a string variable of the form 5*10/20+6 and your program should output the result. The challenge here is first you have the input in string format and secondly you got to follow the BODMAS rules.
Hope this helps someone.
Thank you Anjana for sharing these useful interview questions with us. Do you mind sharing your answers as well?
DeleteHi, Can you share the answer of your 1st question?
DeleteJust figured the solution for Anjana's first question. Hope it helps you guys :)
Deleteimport java.util.Scanner;
public class clockAngles {
public static void main(String []args)
{
Scanner reader = new Scanner(System.in);
System.out.println("Enter the Time (Hr:Min): ");
String time = reader.next();
reader.close();
String[] parts = time.split(":");
String Hour = parts[0];
String Minutes = parts[1];
int hr = Integer.parseInt(Hour);
int min = Integer.parseInt(Minutes);
hr*=5;
if(hr>59) hr=0;
int angle = Math.abs((min-hr)*6); //1 minute on the clock is 6 degrees
System.out.println("The angle between the hour hand and the minute hand is: "+angle+"°");
}
}
First one, maybe this?
Deleteimport java.util.Scanner;
public class ClockAngle {
public static void main(String[] args) {
// TODO Auto-generated method stub
int eachMinuteAngle = 360/60;
int eachHourAngle = 360/12;
Scanner reader = new Scanner(System.in);
System.out.println("Enter hours: ");
int hour = reader.nextInt();
System.out.println("Enter minutes: ");
int minute = reader.nextInt();
reader.close();
//Convert 24h to 12h
if(hour >= 12)
{
hour -= 12;
}
int hourAngle = hour*eachHourAngle;
int minuteAngle = minute*eachMinuteAngle;
System.out.println(Math.abs(hourAngle-minuteAngle));
}
}
Why do you think that i % (3*5) == 0 is right algorithm in FizzBus problem? I am sure, that you should march 3 and 5 simultaneously, then 3 and 5 separately. But 15 is wrong idea. What can you say?
ReplyDeleteWhat is the basic reason for this question?
ReplyDeleteclass demo1
{public static void main(String[] args)
{
final Integer ifour=4;
Integer iRef=4;
final int iLoc = 3;
switch (iRef) {
case 1:
case iLoc:
case 2 * iLoc:
System.out.println("I am not OK.");
default:
System.out.println("You are OK.");
case iRef: //or case iLoc:
System.out.println("It's OK.");
}}}
i have this kind of question
ReplyDeletewrite program to print this
*******
*Some*
*Text *
*Here *
*******
what is the error in code to sort date in ascending order using collection
ReplyDeleteimport java.util.*;
import java.io.*;
import java.lang.*;
class date1
{
public static void main(String args[])
{
List dateList=new ArrayList<>();
dateList.add("02-05-2014");
dateList.add("12-05-2012");
dateList.add("02-02-2014");
dateList.add("02-06-2015");
sortDates(dateList);
for(String sortedDate : dateList)
System.out.println(sortedDate);
}
}
private static sortDates(List dateList)
{
Collections.sort(dateList,new Comparator())
DateFormat dateFormat=new SimpleDateFormat("dd-MM-yyyy");
public int compare(String DATE_1,String DATE_2)
{
try
{
return dateFormat.parse(DATE_1).compareTodateFormat.parse(DATE_2));
}
catch(ParseException e)
{
throw new IlegalArgumentExeption(e);
}
}
});
}
good explanation
ReplyDeletei am a java beginner,i don,t know which database are best with java,java(spring),mysql,sql server,or oracle which one i need to learn.
ReplyDeleteyou can pick mysql or oracle. theres not much difference between them.
DeleteWhere can I find Java development tasks for a job interview?
ReplyDeleteI prepared myself with:
ReplyDelete"Cracking the Coding Interview" by Gayle Laakmann McDowell
"TOP 30 Java Interview Coding Tasks" by Matthew Urban
"Elements of Programming Interviews in Java" by Adnan Aziz, Tsung-Hsien Lee, Amit Prakash
"Coding Interview Questions" by Narasimha Karumanchi
I bought "TOP 30 Java Interview Coding Tasks" by Matthew Urban as well. Really recommend. Good book, all the infos there. Thanks.
Deletereally good works by commenters thank you lot
ReplyDeleteplease let me know the answer. thanks
ReplyDelete1. Filtering Strings
ItemSeparator is a class which filters a given raw input string of the format
"ItemName$$##ItemPrice$$##ItemQuantity", and stores it as these class attributes:
1. name (String)
2. price (Double)
3. quantity (Integer)
Implement the constructor ItemSeparator(String rawInput) as well as the getter methods for all the 3
attributes of the class ItemSeperator:
1. String getName
2. Double getPrice
3. Integer getQuantity