You cannot print array elements directly in Java, you need to use Arrays.toString() or Arrays.deepToString() to print array elements. Use toString() if you want to print a one-dimensional array and use deepToString() method if you want to print a two-dimensional array. Have you tried printing arrays in Java before? What did you do? just passed an array to println() method and expecting it prints its elements? Me too, but surprisingly array despite being Object and providing a length field, doesn't seem overriding the toString() method from java.lang.Object class. All it prints is type@somenumber. This is not at all useful for anyone who is interested in seeing whether an array is empty or not, if not then what elements it has etc.
To print Java array in a meaningful way, you don't need to look further because your very own Collection framework provides lots of array utility methods in java.util.Arrays class. Here we have toString() and deepToString() method to print array in Java.
These methods are overloaded, much like System.out.println() method to accept all primitive types, which means a different method is called if you pass a boolean array, and a different one is called when you print integer array.
The same is true with deepToString(), which is used to print two-dimensional arrays in Java. In this Java array tutorial, we will see examples of a printing string array, integer array, byte array and a two-dimensional array in Java. The rest of them are like that, which means by following these examples, you should be able to print boolean, char, short, float, double and long array by your own.
Arrays class provides a different method to print a two-dimensional array in Java, it’s called toDeepString(). It's capable of printing multi-dimensional array in Java and similar to toDeepEquals() which is used to compare multi-dimensional array in Java. This method is also overloaded and provides 8 + 1 primitive and object versions to accept boolean, byte, short, char, int, long, float, double and Object in Java. Here is an example of how to print a two-dimensional array in Java.
To print Java array in a meaningful way, you don't need to look further because your very own Collection framework provides lots of array utility methods in java.util.Arrays class. Here we have toString() and deepToString() method to print array in Java.
These methods are overloaded, much like System.out.println() method to accept all primitive types, which means a different method is called if you pass a boolean array, and a different one is called when you print integer array.
The same is true with deepToString(), which is used to print two-dimensional arrays in Java. In this Java array tutorial, we will see examples of a printing string array, integer array, byte array and a two-dimensional array in Java. The rest of them are like that, which means by following these examples, you should be able to print boolean, char, short, float, double and long array by your own.
How to Print int array in Java - Examples
In order to print an integer array, all you need to do is call Arrays.toString(int array) method and pass your integer array to it. This method will take care of the printing content of your integer array, as shown below. If you directly pass int array to System.out.println(), you will only see the type of array and a random number.int[] primes = {5, 7, 11, 17, 19, 23, 29, 31, 37}; System.out.println("Prime numbers : " + primes); System.out.println("Real prime numbers : " + Arrays.toString(primes)); //Ok Output: Prime numbers : [I@5eb1404f Real prime numbers : [5, 7, 11, 17, 19, 23, 29, 31, 37]
How to Print byte array in Java
the printing byte array is no different than printing int array, as Arrays class provides an overloaded method toString(byte[] bytes) to print contents of byte array in Java, as shown below. By the way, if you want to print byte array as Hex String then see this link.String random = "In Java programming langue, array is object"; byte[] bytes = random.getBytes(); System.out.println("What is inside bytes : " + bytes); System.out.println("Not visible, check closely .." + Arrays.toString(bytes)); Output What is inside bytes : [B@31602bbc Not visible, check closely ..[73, 110, 32, 74, 97, 118, 97, 32, 112, 114, 111, 103, 114, 97, 109, 109, 105, 110, 103, 32, 108, 97, 110, 97, 103, 117, 101, 44, 32, 97, 114, 114, 97, 121, 32, 105, 115, 32, 111, 98, 106, 101, 99, 116]
How to Print String array in Java
Printing string array in Java is probably the easiest thing to do because Arrays class has another overloaded version of toString() to accept Object. This method calls toString() of Object to get a printable String. This can also be used to print the array of any arbitrary object in Java. The user-defined object must override the toString() method to show something reasonable on the console.String[] buzzwords = {"Java", "Android", "iOS", "Scala", "Python"}; System.out.println("Buzzing .." + buzzwords); System.out.println("Not buzzing? try again : " + Arrays.toString(buzzwords)); Output: Buzzing ..[Ljava.lang.String;@46f5331a Not buzzing? try again : [Java, Android, iOS, Scala, Python]
How to Print Two Dimensional Array in Java
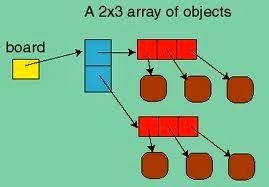
String[][] phones = {{"Apple", "iPhone"}, {"Samsung", "Galaxy"}, {"Sony", "Xperia"}}; System.out.println("Hot phones .. " + phones); System.out.println("Not hot? See again.." + Arrays.deepToString(phones)); Output Hot phones .. [[Ljava.lang.String;@57398044 Not hot? See again..[[Apple, iPhone], [Samsung, Galaxy], [Sony, Xperia]]
Complete Java Program to Print Array in Java
This is the full Java code of print different types of arrays in Java. As explained in this article, it prints integer, String, byte, and two-dimensional array using toString() and deepToString() method of java.util.Arrays class.You can copy-paste this program in your Java IDE and run it. Don't need any third-party libraries.
That's all about how to print array in Java. We have learned how to print objects of an array, instead of an array object, which is nothing but a hashCode. I really hope that Java should add a toString() in Array, instead of providing Arrays.toString(), don't know when they chose other parts.
import java.util.Arrays; /** * Java Program to print arrays in Java. We will learn how to print String, int, * byte and two dimensional arrays in Java by using toString() and * deepToString() method of Arrays class. * * @author http://java67.blogspot.com */ public class PrintArrayInJava{ public static void main(String args[]) { // Example 1 : print int array in Java int[] primes = {5, 7, 11, 17, 19, 23, 29, 31, 37}; System.out.println("Prime numbers : " + primes); // Not OK System.out.println("Real prime numbers : " + Arrays.toString(primes)); //Ok // Example 2 : print String array in Java String[] buzzwords = {"Java", "Android", "iOS", "Scala", "Python"}; System.out.println("Buzzing .." + buzzwords); System.out.println("Not buzzing? try again : " + Arrays.toString(buzzwords)); // Example 3 : print two dimensional array in Java String[][] phones = {{"Apple", "iPhone"}, {"Samsung", "Galaxy"}, {"Sony", "Xperia"}}; System.out.println("Hot phones .. " + phones); System.out.println("Not hot? See again.." + Arrays.deepToString(phones)); // Example 4 : print byte array in Java String random = "In Java programming langue, array is object"; byte[] bytes = random.getBytes(); System.out.println("What is inside bytes : " + bytes); System.out.println("Not visible, check closely .." + Arrays.toString(bytes)); } } Output: Prime numbers : [I@5eb1404f Real prime numbers : [5, 7, 11, 17, 19, 23, 29, 31, 37] Buzzing ..[Ljava.lang.String;@46f5331a Not buzzing? try again : [Java, Android, iOS, Scala, Python] Hot phones .. [[Ljava.lang.String;@57398044 Not hot? See again..[[Apple, iPhone], [Samsung, Galaxy], [Sony, Xperia]] What is inside bytes : [B@31602bbc Not visible, check closely ..[73, 110, 32, 74, 97, 118, 97, 32, 112, 114, 111, 103, 114, 97, 109, 109, 105, 110, 103, 32, 108, 97, 110, 97, 103, 117, 101, 44, 32, 97, 114, 114, 97, 121, 32, 105, 115, 32, 111, 98, 106, 101, 99, 116]
And, here is a nice summary of how to print array in Java:
Nevertheless, toString() and toDeepString() from java.util.Arrays class is sufficient to print any kind of one-dimensional and two-dimensional array in Java. Though special care needs to take, while printing byte arrays, which requires byte to be encoded in Hex string.
In "How to Print byte array in Java", how to print the original string from byte[] ??
ReplyDeleteHello @samiulla, you can convert byte array to String using new String(). If its coming from other host or encoded in some other encoding other than platform's default, provide that encoding e.g. "UTF-8" to String constructor.
DeleteI have a JAXBElement element 'baseData' which has some base64 data in it. Am trying to read this using (new String(baseData.getData().getValue())). But all I get is non-readable characters. If I use just (baseData.getData().getValue()), I get the offset address. Am just lost since two days. Can you please help me with this? I want to read the Base64 data and print it.
DeleteI tried to find the method toDeepEquals() but success. there is a method Arrays.deepEquals().
ReplyDeletePlease Update the articles for further/next reader. if i am right.
Thanks and Regards
Shesh Narayan Chakrapani.
Awesome example's
ReplyDeleteHow print s2 :
ReplyDeleteArray [] s1 = {"a",1};
Array [] s2 = { "b" , s1};
System.out.println("... " + s2 ?